What Is MongoDB, Tutorial On MongoDB, CURD Operation In MongoDB
Hello guys, welcome back to our blog. In this article, we will discuss what is MongoDB, why to use mongo_db, tutorials on MongoDB, CURD operation, query operations, aggregation in MongoDB, etc.
If you have any electrical, electronics, and computer science doubts, then ask questions. You can also catch me on Instagram – CS Electrical & Electronics.
Also, read:
- Top 50 IDE For Developers, IDE For Programmers, IDE Tools For Engineer.
- Top 10 YouTube Channels For Computer Science Engineers.
- SQL Tutorial For Data Analytics, Tutorial On SQL, SQL Operations.
What Is MongoDB
Mongo DB is a cross-platform, document-oriented database that provides:
- High performance.
- High availability.
- Easy scalability.
MongoDB works on the concept of collection and document. MongoDB is a NoSQL program. It uses JSON format. You can download MongoDB from their website Mongodb.com. MongoDB is famous among new developers due to its flexibility and ease of usage. Even though it’s easy to use it still offers all the capabilities required to meet the complex needs of modern applications. A lot of developers like Mongo because it stores data or all of its documents in JSON.
Why use MongoDB
- All modern applications deal with huge data and Mongo DB can handle it.
- Development with ease is possible with Mongo DB.
- Flexibility in deployment.
- Rich Queries.
- Older database systems may not be compatible with the design.
Mongo DB vs RDBMS
RDBMS | MongoDB | |
Database | -> | Database |
Table, View | -> | Collection |
Row | -> | Document (BSON) |
Column | -> | Field |
Index | -> | Index |
Join | -> | Embedded Document |
Foreign Key | -> | Reference |
Partition | -> | Shard |
Mongo DB Architecture
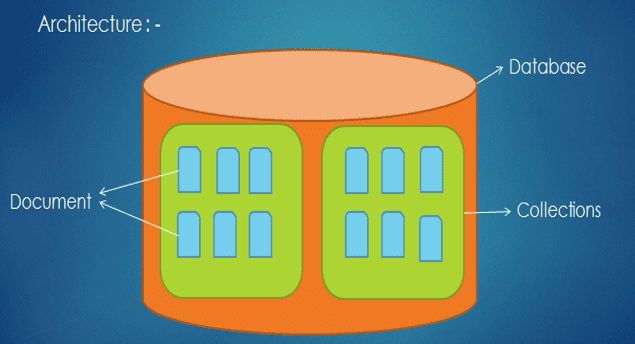
Data Model
- Document-Based (max 16 MB)
- Documents are in BSON format, consisting of field-value pairs.
- Each document stored in a collection
JSON
- MongoDB is a NoSQL program and uses JSON format.
- “JavaScript Object Notation”.
- Easy for humans to write/read, easy for computers to parse/generate.
- Objects can be nested.
- Built on: name/value pairs and Ordered list of values.
BSON
- “Binary JSON”: Binary-encoded serialization of JSON-like docs.
- Also allows “referencing”.
- The embedded structure reduces the need for joins.
- Goals: Lightweight, Traversable, and Efficient (decoding and encoding).
BSON Example
{
"_id" : "37010"
"city" : "ADAMS",
"pop" : 2660,
"state" : "TN",
“councilman” : {
name: “John Smith”
address: “13 Scenic Way”
}
}
BSON Types
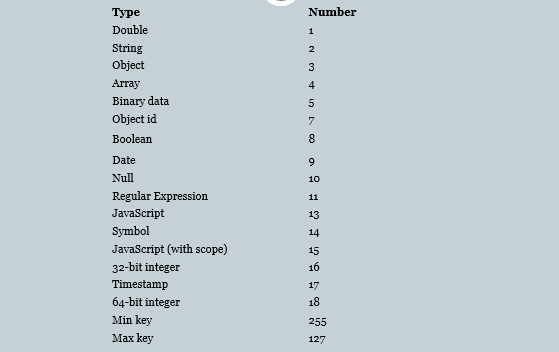
CURD operations in Mongo DB
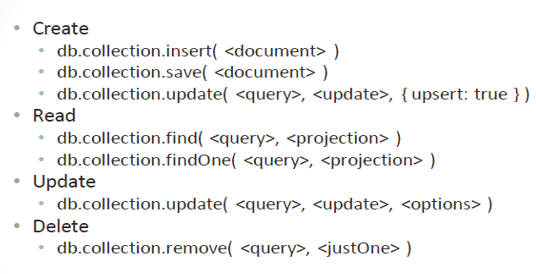
MongoDB Tutorial
I will cover these topics, such as:
- Step-up database
- CURD operations
- Query operations
- Aggregation in MongoDB
Read the article on how to install MongoDB and step up data – Click Here.
Once you install MongoDB, then open a command window and type: C:\Users\HP>mongo
C:\Users\HP>mongo
MongoDB shell version v4.4.5
connecting to: mongodb://127.0.0.1:27017/?compressors=disabled&gssapiServiceName=mongodb
Implicit session: session { "id" : UUID("1a64f23d-1054-4d83-b2f1-0f0e537fc54e") }
MongoDB server version: 4.4.5
---
The server generated these startup warnings when booting:
2021-05-15T14:14:17.190+05:30: Access control is not enabled for the database. Read and write access to data and configuration is unrestricted
---
---
Enable MongoDB's free cloud-based monitoring service, which will then receive and display
metrics about your deployment (disk utilization, CPU, operation statistics, etc).
The monitoring data will be available on a MongoDB website with a unique URL accessible to you
and anyone you share the URL with. MongoDB may use this information to make product
improvements and to suggest MongoDB products and deployment options to you.
To enable free monitoring, run the following command: db.enableFreeMonitoring()
To permanently disable this reminder, run the following command: db.disableFreeMonitoring()
---
>
This will connect MongoDB and now type “show dbs”, it will display all the databases that you have created.
> show dbs
admin 0.000GB
config 0.000GB
local 0.000GB
mydata 0.000GB
mydbs 0.000GB
myfirstdatabase 0.000GB
>
Now, I will create a new database of cricket players. For that use <namefordatabse>.
> use mydbplayer
switched to db mydbplayer
Now, new database is created of name mydbplayer. Now, we will add cricket player details. For that type: db.cricketplayer.insert({“Name”:”Virat”, “Age”: 34, “Id” : 1, “Team”:”India”, “Role”:”Batsmen”});
> db.cricketplayer.insert({"Name":"Virat", "Age": 34, "Id" : 1, "Team":"India", "Role":"Batsmen"});
WriteResult({ "nInserted" : 1 })
>
I added one cricket player’s details to the database. Now, I will add four more details about cricket players.
> db.cricketplayer.insert({"Name":"Dhoni", "Age": 36, "Id": 2, "Team":"India", "Role":"Batsmen and wicket keep"});
WriteResult({ "nInserted" : 1 })
> db.cricketplayer.insert({"Name":"AB devilliers", "Age": 31, "Id": 3, "Team":"south africa", "Role":"Batsmen"});
WriteResult({ "nInserted" : 1 })
> db.cricketplayer.insert({"Name":"Rohit", "Age": 28, "Id": 4, "Team":"India", "Role":"Batsmen"});
WriteResult({ "nInserted" : 1 })
> db.cricketplayer.insert({"Name":"Kraigg", "Age": 26, "Id": 5, "Team":"West Indies", "Role":"Batsmen and bowler"});
WriteResult({ "nInserted" : 1 })
>
I have added five cricket players’ details to the database. Now, I will perform CRUD operations: Create, Read, Update, Delete.
First, I will display all cricketers details. For that, type: db.cricketplayer.find({});
> db.cricketplayer.find({});
{ "_id" : ObjectId("60b1ef1f5eafcf6fb31c8f57"), "Name" : "Virat", "Age" : 34, "Id" : 1, "Team" : "India", "Role" : "Batsmen" }
{ "_id" : ObjectId("60b1f2095eafcf6fb31c8f58"), "Name" : "Dhoni", "Age" : 36, "Id" : 2, "Team" : "India", "Role" : "Batsmen and wicket keep" }
{ "_id" : ObjectId("60b1f2525eafcf6fb31c8f59"), "Name" : "AB devilliers", "Age" : 31, "Id" : 3, "Team" : "south africa", "Role" : "Batsmen" }
{ "_id" : ObjectId("60b1f4e15eafcf6fb31c8f5d"), "Name" : "Rohit", "Age" : 28, "Id" : 4, "Team" : "India", "Role" : "Batsmen" }
{ "_id" : ObjectId("60b1f5085eafcf6fb31c8f5e"), "Name" : "Kraigg", "Age" : 26, "Id" : 5, "Team" : "West Indies", "Role" : "Batsmen and bowler" }
>
Now, I will delete or remove one cricket player details and for that type: db.cricketplayer.remove({“Id”:5});
> db.cricketplayer.remove({"Id":5});
WriteResult({ "nRemoved" : 1 })
>
Details of Id:5 player is removed or deleted, Now, I will display details again.
> db.cricketplayer.find({});
{ "_id" : ObjectId("60b1ef1f5eafcf6fb31c8f57"), "Name" : "Virat", "Age" : 34, "Id" : 1, "Team" : "India", "Role" : "Batsmen" }
{ "_id" : ObjectId("60b1f2095eafcf6fb31c8f58"), "Name" : "Dhoni", "Age" : 36, "Id" : 2, "Team" : "India", "Role" : "Batsmen and wicket keep" }
{ "_id" : ObjectId("60b1f2525eafcf6fb31c8f59"), "Name" : "AB devilliers", "Age" : 31, "Id" : 3, "Team" : "south africa", "Role" : "Batsmen" }
{ "_id" : ObjectId("60b1f4e15eafcf6fb31c8f5d"), "Name" : "Rohit", "Age" : 28, "Id" : 4, "Team" : "India", "Role" : "Batsmen" }
>
Now, I will update the age of Virat from 34 to 32. For that type: db.cricketplayer.update({“Name”:”Virat”},{$set:{“Age”:32}});
> db.cricketplayer.update({"Name":"Virat"},{$set:{"Age":32}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
Now, I will display all cricketer’s details again and you can see the age of Virat will be changed from 34 to 32.
> db.cricketplayer.find({});
{ "_id" : ObjectId("60b1ef1f5eafcf6fb31c8f57"), "Name" : "Virat", "Age" : 32, "Id" : 1, "Team" : "India", "Role" : "Batsmen" }
{ "_id" : ObjectId("60b1f2095eafcf6fb31c8f58"), "Name" : "Dhoni", "Age" : 36, "Id" : 2, "Team" : "India", "Role" : "Batsmen and wicket keep" }
{ "_id" : ObjectId("60b1f2525eafcf6fb31c8f59"), "Name" : "AB devilliers", "Age" : 31, "Id" : 3, "Team" : "south africa", "Role" : "Batsmen" }
{ "_id" : ObjectId("60b1f4e15eafcf6fb31c8f5d"), "Name" : "Rohit", "Age" : 28, "Id" : 4, "Team" : "India", "Role" : "Batsmen" }
>
Now, I will insert the id:5 player details again that I have deleted. For that type:
> db.cricketplayer.insert({"Name":"Kraigg", "Age": 26, "Id": 5, "Team":"West Indies", "Role":"Batsmen and bowler"});
WriteResult({ "nInserted" : 1 })
>
Now, I will display all player details again. For that type:
> db.cricketplayer.find({});
{ "_id" : ObjectId("60b1ef1f5eafcf6fb31c8f57"), "Name" : "Virat", "Age" : 32, "Id" : 1, "Team" : "India", "Role" : "Batsmen" }
{ "_id" : ObjectId("60b1f2095eafcf6fb31c8f58"), "Name" : "Dhoni", "Age" : 36, "Id" : 2, "Team" : "India", "Role" : "Batsmen and wicket keep" }
{ "_id" : ObjectId("60b1f2525eafcf6fb31c8f59"), "Name" : "AB devilliers", "Age" : 31, "Id" : 3, "Team" : "south africa", "Role" : "Batsmen" }
{ "_id" : ObjectId("60b1f4e15eafcf6fb31c8f5d"), "Name" : "Rohit", "Age" : 28, "Id" : 4, "Team" : "India", "Role" : "Batsmen" }
{ "_id" : ObjectId("60b1f7fd5eafcf6fb31c8f5f"), "Name" : "Kraigg", "Age" : 26, "Id" : 5, "Team" : "West Indies", "Role" : "Batsmen and bowler" }
>
This was about CRUD operation, I have performed read operation, update operation, create or insert operation, and delete or remove operation. Now, we will move to query operations.
Query Operators
Name | Description |
$eq | Matches values that are equal to a specified value. |
$gt, $gte | Matches values that are greater than ( or equal to a specified value). |
$lt, $lte | Matches values less than or (equal to) a specified value. |
$ne | Matches values that are not equal to a specified value. |
$in | Matches any of the values specified in an array. |
$nin | Matches none of the values specified in an array. |
$or | Joins query clauses with a logical OR returns all. |
$and | Join query clauses with a logical AND. |
$not | Inverts the effect of a query expression. |
Now, I will perform some query operations. I will display the cricket player names whose age is less then 30 years. For that type: db.cricketplayer.find({“Age”:{“$lt”:30}});
> db.cricketplayer.find({"Age":{"$lt":30}});
{ "_id" : ObjectId("60b1f4e15eafcf6fb31c8f5d"), "Name" : "Rohit", "Age" : 28, "Id" : 4, "Team" : "India", "Role" : "Batsmen" }
{ "_id" : ObjectId("60b1f7fd5eafcf6fb31c8f5f"), "Name" : "Kraigg", "Age" : 26, "Id" : 5, "Team" : "West Indies", "Role" : "Batsmen and bowler" }
>
Now, I will display cricket player names whose age is greater then 30 years. For that type: db.cricketplayer.find({“Age”:{“$gt”:30}});
> db.cricketplayer.find({"Age":{"$gt":30}});
{ "_id" : ObjectId("60b1ef1f5eafcf6fb31c8f57"), "Name" : "Virat", "Age" : 32, "Id" : 1, "Team" : "India", "Role" : "Batsmen" }
{ "_id" : ObjectId("60b1f2095eafcf6fb31c8f58"), "Name" : "Dhoni", "Age" : 36, "Id" : 2, "Team" : "India", "Role" : "Batsmen and wicket keep" }
{ "_id" : ObjectId("60b1f2525eafcf6fb31c8f59"), "Name" : "AB devilliers", "Age" : 31, "Id" : 3, "Team" : "south africa", "Role" : "Batsmen" }
>
Now, I will perform OR operation and for that type: db.cricketplayer.find({$or: [{“Age”:{$lt:30}}, {“Role”:”Batsmen”}]});
> db.cricketplayer.find({$or: [{"Age":{$lt:30}}, {"Role":"Batsmen"}]});
{ "_id" : ObjectId("60b1ef1f5eafcf6fb31c8f57"), "Name" : "Virat", "Age" : 32, "Id" : 1, "Team" : "India", "Role" : "Batsmen" }
{ "_id" : ObjectId("60b1f2525eafcf6fb31c8f59"), "Name" : "AB devilliers", "Age" : 31, "Id" : 3, "Team" : "south africa", "Role" : "Batsmen" }
{ "_id" : ObjectId("60b1f4e15eafcf6fb31c8f5d"), "Name" : "Rohit", "Age" : 28, "Id" : 4, "Team" : "India", "Role" : "Batsmen" }
{ "_id" : ObjectId("60b1f7fd5eafcf6fb31c8f5f"), "Name" : "Kraigg", "Age" : 26, "Id" : 5, "Team" : "West Indies", "Role" : "Batsmen and bowler" }
>
This will display players details whose age is less then 30 and players whose role is batsmen.
Now, I will perform AND operation for the same and for that type: db.cricketplayer.find({$and: [{“Age”:{$lt:30}}, {“Role”:”Batsmen”}]});
> db.cricketplayer.find({$and: [{"Age":{$lt:30}}, {"Role":"Batsmen"}]});
{ "_id" : ObjectId("60b1f4e15eafcf6fb31c8f5d"), "Name" : "Rohit", "Age" : 28, "Id" : 4, "Team" : "India", "Role" : "Batsmen" }
>
Now, I will perform $in operation, I will display players details whose age is 32 to 36. It is just like a OR operation For that type: db.cricketplayer.find({“Age”: {$in:[32,35]}});
> db.cricketplayer.find({"Age": {$in:[32,35]}});
{ "_id" : ObjectId("60b1ef1f5eafcf6fb31c8f57"), "Name" : "Virat", "Age" : 32, "Id" : 1, "Team" : "India", "Role" : "Batsmen" }
> db.cricketplayer.find({"Age": {$in:[32,36]}});
{ "_id" : ObjectId("60b1ef1f5eafcf6fb31c8f57"), "Name" : "Virat", "Age" : 32, "Id" : 1, "Team" : "India", "Role" : "Batsmen" }
{ "_id" : ObjectId("60b1f2095eafcf6fb31c8f58"), "Name" : "Dhoni", "Age" : 36, "Id" : 2, "Team" : "India", "Role" : "Batsmen and wicket keep" }
>
This was about query operations in MongoDB. Remaining operations you can try by yourself.
Aggregation in Mongo DB
Expression | Description | Example |
$sum | Sums up the defined value from all documents in the collection. | db.mycol.aggregate([{$group: {_id : “$by_user”, num_tutorial : {$sum : “$likes”}}}]) |
$avg | Calculates the average of all given values from all documents in the collection. | db.mycol.aggregate([{$group: {_id : “$by_user”, num_tutorial : {$avg : “$likes”}}}]) |
$min | Gets the minimum of the corresponding values from all documents in the collection. | db.mycol.aggregate([{$group :{_id : “$by_user”, num_tutorial : {$min : “$likes”}}}]) |
$max | Gets the maximum of the corresponding values from all documents in the collection. | db.mycol.aggregate([{$group :{_id : “$by_user”, num_tutorial : {$max : “$likes”}}}]) |
You can try these aggregation operations.
Limitations of Mongo DB
- No referential integrity.
- The high degree of demoralization means updating something in many places instead of one.
Conclusions
- Very rapid development, open-source.
- Useful when working with a huge quantity of data when the data’s nature does not require a relational model.
- Mongo DB is fast.
This was about “What Is MongoDB“. I hope this article may help you all a lot. Thank you for reading.
Also, read:
- 100+ C Programming Projects With Source Code, Coding Projects Ideas
- 1000+ Interview Questions On Java, Java Interview Questions, Freshers
- App Developers, Skills, Job Profiles, Scope, Companies, Salary
- Applications Of Artificial Intelligence (AI) In Renewable Energy
- Applications Of Artificial Intelligence, AI Applications, What Is AI
- Applications Of Data Structures And Algorithms In The Real World
- Array Operations In Data Structure And Algorithms Using C Programming
- Artificial Intelligence Scope, Companies, Salary, Roles, Jobs
Author Profile
- Chetu
- Interest's ~ Engineering | Entrepreneurship | Politics | History | Travelling | Content Writing | Technology | Cooking
Latest entries
All PostsApril 29, 2024Top 11 Free Courses On Battery For Engineers With Documents
All PostsApril 19, 2024What Is Vector CANoe Tool, Why It Is Used In The Automotive Industry
All PostsApril 13, 2024What Is TCM, Transmission Control Module, Working, Purpose,
All PostsApril 12, 2024Top 100 HiL hardware in loop Interview Questions With Answers For Engineers