How To Create Multiple Files And Makefile In VS Code On Windows
Hello guys, welcome back to my blog. In this article, I will discuss how to create multiple files and makefile in VS code on windows, makefile on visual studio code, multiple files in visual studio code, etc.
If you have any doubts related to electrical, electronics, and computer science, then ask question. You can also catch me @ Instagram – Chetan Shidling.
Also, read:
- What Is Unit Testing, Why Unit Testing Is Required, Its Importance
- What Is Linux, Applications Of Linux, Why To Learn Linux
- Top 10 Applications Of Blockchain In Different Domains, Technology
Multiple Files And Makefile In VS Code
Multiple files are essential to make programmers’ lives more relaxed, but I believe this gives you a greater feel for what “large applications” can cause. I have been recognized to write large applications in various different files. They state it will run faster and debugging with multiple files is much easy.
Why makefile is required?
Makefile in multiple programs is required to run all files. You require a file called a makefile to state make what to do. Most often, the makefile says make how to compile and link a multiple code or program. It defines a set of tasks to be executed.
Multiple File On Electricity Bill In C
I will take the example of an electricity bill, here I will create two .c files and two .h files. Before creating the makefile, make sure that you have installed visual studio code, extensions, and gcc.
01. Create a folder and give it any name.
02. Inside the folder create four files, two for .c files and two for .h files. (.h means I am creating my own header file).
03. After creating files, I have written code.
04. Then create a new file and give it a name called “MakeFile”.
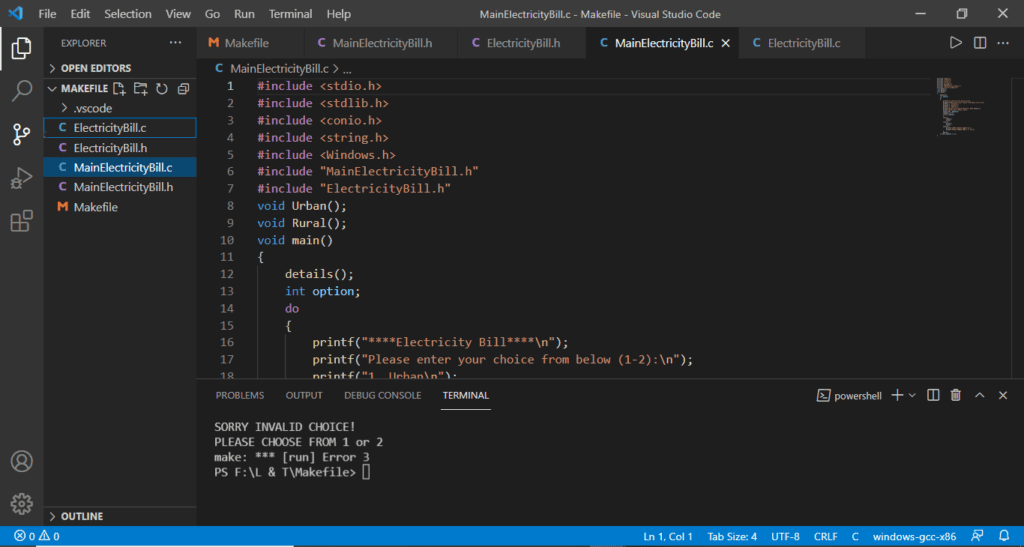
05. In the above image you can see a total there are five files.
06. Let me share my code with you.
a. MainElectricityBill.h
#ifndef __MainElectricityBill_H__
#define __MainElectricityBill_H__
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
#include<string.h>
#include<Windows.h>
struct ElectricBill
{
char area[6];
char name[15];
int meternumber;
int unitsconsumed;
char email[15];
char address[100];
int phonenumber[10];
};
struct ElectricBill B;
#endif
b. MainElectricityBill.c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <string.h>
#include <Windows.h>
#include "MainElectricityBill.h"
#include "ElectricityBill.h"
void Urban();
void Rural();
void main()
{
details();
int option;
do
{
printf("****Electricity Bill****\n");
printf("Please enter your choice from below (1-2):\n");
printf("1. Urban\n");
printf("2. Rural\n");
printf("3. EXIT\n");
printf("Electricity Board Helpline: 8435 2340\n");
printf("Enter your choice :\n");
scanf("%d", &option);
system("cls");
switch (option)
{
case 1:
Urban();
break;
case 2:
Rural();
break;
default:
printf("SORRY INVALID CHOICE!\n");
printf("PLEASE CHOOSE FROM 1 or 2\n");
}
getch();
} while (option != 3);
}
c. ElectricityBill.h
#ifndef __ElectricityBill_H__
#define __ElectricityBill_H__
void Urban();
void Rural();
#endif
d. ElectricityBill.c
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
#include<string.h>
#include<Windows.h>
#include"MainElectricityBill.h"
void details()
{
printf("Enter your area name:\n");
scanf("%s", B.area);
printf("Enter your name:\n");
scanf("%s", B.name);
printf("Enter your Meter Number:\n");
scanf("%d", &B.meternumber);
printf("Enter units consumed:\n");
scanf("%d", &B.unitsconsumed);
printf("Enter email ID :\n");
scanf("%s", B.email);
printf("Enter the permanent address :\n");
scanf("%s", B.address);
printf("Enter the phone number : \n");
scanf("%s", B.phonenumber);
}
void Urban()
{
int amount=0;
B.unitsconsumed;
if(B.unitsconsumed <=30 && B.unitsconsumed >= 0)
{
amount = B.unitsconsumed * 3.25; //3.25 is rupees
}
else if(B.unitsconsumed >= 31 && B.unitsconsumed <= 100)
{
amount = B.unitsconsumed * 4.70;
}
else if(B.unitsconsumed >= 101 && B.unitsconsumed <= 200)
{
amount = B.unitsconsumed * 6.25;
}
else
{
amount = B.unitsconsumed * 7.30;
}
printf("****Electricity Bill****\n\n");
printf("Name : %s\n", B.name);
printf("In Urban, your electricity bill is: %d\n", amount);
printf("Units you consumed per month: %d\n", B.unitsconsumed);
}
void Rural()
{
int amount=0;
B.unitsconsumed;
if(B.unitsconsumed <=30 && B.unitsconsumed >= 0)
{
amount = B.unitsconsumed * 3.15; //3.25 is rupees
}
else if(B.unitsconsumed >= 31 && B.unitsconsumed <= 100)
{
amount = B.unitsconsumed * 4.40;
}
else if(B.unitsconsumed >= 101 && B.unitsconsumed <= 200)
{
amount = B.unitsconsumed * 5.95;
}
else
{
amount = B.unitsconsumed * 6.80;
}
printf("****Electricity Bill****\n\n");
printf("Name : %s\n", B.name);
printf("In Rural, your electricity bill is: %d\n", amount);
printf("Units you consumed per month: %d\n", B.unitsconsumed);
}
e. MakeFile
If you want to add your file name, then do changes in SRC,
SRC = ElectricityBill.c\
MainElectricityBill.c
# Name of the project
PROJECT_NAME = Calculator
# Output directory
BUILD = build
# All source code files
SRC = ElectricityBill.c\
MainElectricityBill.c
# Project Output name
PROJECT_OUTPUT = $(BUILD)/$(PROJECT_NAME).out
# Default target built
$(PROJECT_NAME):all
# Run the target even if the matching name exists
.PHONY: run clean test doc all
all: $(SRC) $(BUILD)
gcc $(SRC) $(INC) -o $(PROJECT_OUTPUT).out
# Call `make run` to run the application
run:$(PROJECT_NAME)
./$(PROJECT_OUTPUT).out
# Document the code using Doxygen
#doc:
# make -C ./documentation
# Build and run the unit tests
test:$(BUILD)
gcc $(TEST_SRC) $(INC) -o $(TEST_OUTPUT)
./$(TEST_OUTPUT)
#Coverage
coverageCheck:$(BUILD)
g++ -fprofile-arcs -ftest-coverage -fPIC -O0 $(TEST_SRC) $(INC) -o $(TEST_OUTPUT)
./$(TEST_OUTPUT)
# Remove all the built files, invoke by `make clean`
clean:
rm -rf $(BUILD) $(DOCUMENTATION_OUTPUT)
# Create new build folder if not present
$(BUILD):
mkdir build
07. To run files. Open terminal and type >>make.

08. After running, a build file will be generated.
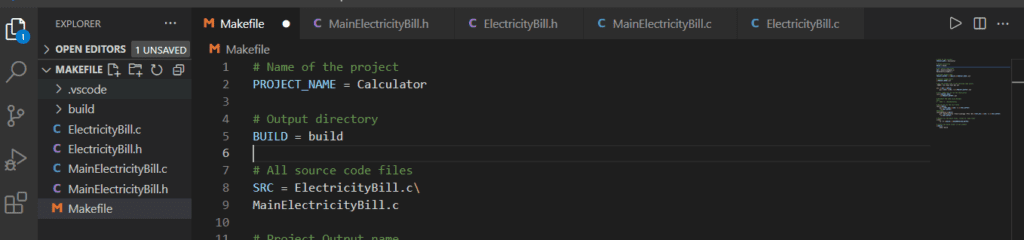
09. Now in terminal, type >> make run. The code will run and enter input and you will get proper results.
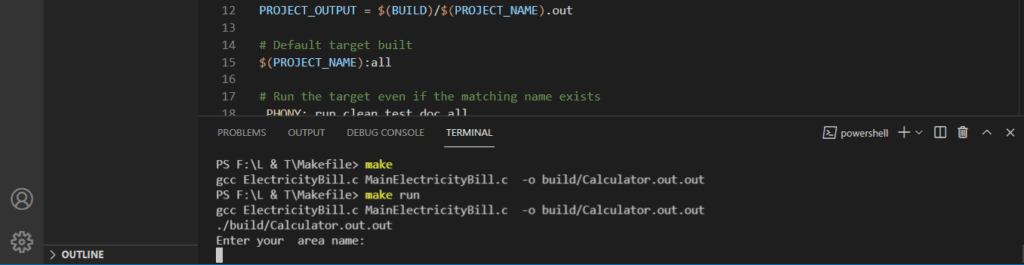
Output:
Enter your area name:
Jawahar
Enter your name:
Chetan
Enter your Meter Number:
12
Enter units consumed:
234
Enter email ID :
abc@gmail.com
Enter the permanent address :
MG
Enter the phone number :
1234567890
****Electricity Bill****
Please enter your choice from below (1-2):
1. Urban
2. Rural
3. EXIT
Electricity Board Helpline: 8435 2340
Enter your choice :
1
Name : Chetan
In Urban, your electricity bill is: 1708 rupees
Units you consumed per month: 234
I hope this article “How To Create Multiple Files And Makefile In VS Code On Windows” may help you all a lot. Thank you for reading “How To Create Multiple Files And Makefile In VS Code On Windows”.
Also, read:
- 100+ C Programming Projects With Source Code, Coding Projects Ideas
- 1000+ Interview Questions On Java, Java Interview Questions, Freshers
- App Developers, Skills, Job Profiles, Scope, Companies, Salary
- Applications Of Artificial Intelligence (AI) In Renewable Energy
- Applications Of Artificial Intelligence, AI Applications, What Is AI
- Applications Of Data Structures And Algorithms In The Real World
- Array Operations In Data Structure And Algorithms Using C Programming
- Artificial Intelligence Scope, Companies, Salary, Roles, Jobs
Author Profile
- Chetu
- Interest's ~ Engineering | Entrepreneurship | Politics | History | Travelling | Content Writing | Technology | Cooking
Latest entries
All PostsApril 29, 2024Top 11 Free Courses On Battery For Engineers With Documents
All PostsApril 19, 2024What Is Vector CANoe Tool, Why It Is Used In The Automotive Industry
All PostsApril 13, 2024What Is TCM, Transmission Control Module, Working, Purpose,
All PostsApril 12, 2024Top 100 HiL hardware in loop Interview Questions With Answers For Engineers